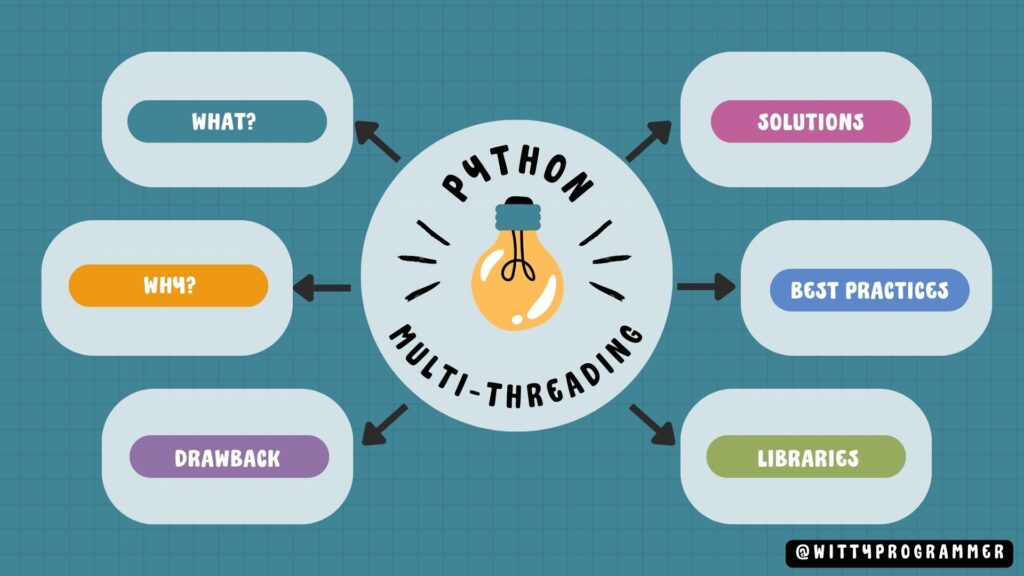
Introduction
Python, known for its simplicity and readability, offers a threading module that allows developers to achieve concurrency. Although Python’s Global Interpreter Lock (GIL) prevents true parallelism, multithreading still offers advantages in certain scenarios. In this article, we’ll delve into the fundamentals of Python multithreading, its benefits, limitations, and best practices to employ in your projects.
Understanding Multithreading
Multithreading involves running multiple threads concurrently within a single process. Threads represent independent sequences of instructions that can be scheduled and executed simultaneously. Python’s threading module provides the necessary tools for creating and managing threads.
Benefits of Multithreading
- Concurrency: Python multithreading enables the execution of multiple tasks concurrently, making efficient use of available resources and enhancing application responsiveness. It is particularly useful for handling I/O-bound tasks, where waiting for external resources can be offloaded to separate threads.
- Code Organization: By employing multithreading, you can compartmentalize different responsibilities or tasks within your application, resulting in better code organization, readability, and maintainability. This approach allows for a structured and manageable codebase.
- Asynchronous Operations: While Python threads do not achieve true parallelism due to the GIL, they can be effectively combined with asynchronous programming techniques like async/await and the asyncio module. By leveraging threads with asynchronous code, you can create a cooperative multitasking environment and effectively handle I/O-bound operations.
Example: Python Multi-Threading
import threading
import requests
# Function to download a file
def download_file(url, filename):
response = requests.get(url)
with open(filename, 'wb') as file:
file.write(response.content)
print(f"Downloaded {filename}")
# List of files to download
files = [
{'url': 'http://ipv4.download.thinkbroadband.com/5MB.zip', 'filename': 'file1.txt'},
{'url': 'http://ipv4.download.thinkbroadband.com/5MB.zip', 'filename': 'file2.txt'},
{'url': 'http://ipv4.download.thinkbroadband.com/5MB.zip', 'filename': 'file3.txt'},
]
# Create a thread for each file
threads = []
for file in files:
url = file['url']
filename = file['filename']
thread = threading.Thread(target=download_file, args=(url, filename))
threads.append(thread)
thread.start()
# Wait for all threads to finish
for thread in threads:
thread.join()
# Output:
# Downloaded file1.txt
# Downloaded file2.txt
# Downloaded file3.txt
Limitations and Considerations
- GIL Limitation: Python’s GIL restricts the true parallel execution of threads for CPU-bound tasks. Consequently, multithreading in Python is most beneficial for I/O-bound operations or when used in conjunction with asynchronous programming.
- Shared State and Synchronization: When multiple threads concurrently access and modify shared data, race conditions, and data inconsistencies may occur. To ensure thread safety and prevent conflicts, proper synchronization mechanisms such as locks, semaphores, and condition variables must be employed.
Key Facts
- GIL Limitation: The GIL allows only one thread to execute Python bytecodes at a time, even on multi-core systems. As a result, threads in Python are more suitable for I/O-bound operations, where they can release the GIL during I/O operations, allowing other threads to run.
- Improved Responsiveness: Multithreading can enhance the responsiveness of applications by allowing concurrent execution of tasks. While each individual thread may not run in parallel, the interleaved execution can provide a sense of concurrency.
- I/O-Bound Efficiency: For I/O-bound tasks such as network requests or file operations, Python threads can be effective. While one thread is waiting for I/O to complete, other threads can continue executing, maximizing the utilization of available resources.
- Limited CPU-Bound Performance: In CPU-bound scenarios where tasks require extensive computational work, multithreading in Python may not provide performance improvements due to the GIL. In fact, it can even introduce some overhead due to the GIL’s synchronization mechanisms.
- Combining with Asynchronous Programming: Python’s
asyncio
module, combined with multithreading, can be a powerful combination. By utilizing asynchronous programming techniques and non-blocking I/O operations, the GIL’s limitations can be mitigated, enabling efficient execution of I/O-bound tasks concurrently.
Best Practices for Python Multithreading
- Identify Appropriate Use Cases: Evaluate your application’s requirements to determine if multithreading is suitable. It works best for I/O-bound tasks, parallelizing independent operations, or when combined with asynchronous programming.
- Utilize Thread Pool Executors: Instead of explicitly creating threads, consider using thread pool executors from the concurrent.futures module. Thread pools efficiently manage a fixed number of worker threads that can handle multiple tasks.
- Minimize CPU-Bound Operations: To avoid contention with the GIL, reduce or eliminate CPU-bound computations within threads. For CPU-intensive tasks, alternative approaches such as multiprocessing or libraries that release the GIL (e.g., NumPy, Numba) may be more suitable.
- Ensure Proper Synchronization: Implement synchronization mechanisms when accessing shared resources to maintain thread safety. Acquire locks or use higher-level synchronization primitives like queues and condition variables to prevent race conditions and maintain data integrity.
- Profile and Optimize: Benchmark and profile your multithreaded code to identify potential bottlenecks or areas for optimization. Measure the performance gains achieved through concurrency and ensure they align with your expectations.
- Selection of Libraries: When it comes to releasing the Global Interpreter Lock (GIL) in Python, there are several libraries and frameworks available that can help you achieve parallelism and improved performance for CPU-bound tasks. These libraries and frameworks are widely used in production-level coding and have proven to be effective in releasing the GIL to achieve parallelism and improved performance for CPU-bound tasks. Here are some notable options:
- NumPy: NumPy is a fundamental library for numerical computing in Python. It provides a multi-dimensional array object and a collection of functions for efficient mathematical operations. Many NumPy operations release the GIL, allowing for parallelism and improved performance.
- Pandas: Pandas is a powerful library for data manipulation and analysis. It builds upon NumPy and provides data structures like DataFrames for handling structured data. Pandas operations that involve large datasets can release the GIL, leading to faster execution.
- Dask: Dask is a flexible library for parallel computing in Python. It provides advanced parallelism and distributed computing capabilities by integrating with other libraries like NumPy, Pandas, and scikit-learn. Dask allows you to scale computations across multiple cores or even distributed systems.
- Cython: Cython is a superset of Python that allows you to write C extensions with Python-like syntax. It provides a way to release the GIL explicitly when needed, enabling efficient parallelism for CPU-bound tasks. Cython code can be compiled to C or C++ and then integrated into your Python project.
- multiprocessing: Although not a library for releasing the GIL, the
multiprocessing
module in the Python standard library allows you to leverage multiple processes instead of threads for parallel execution. Each process runs in a separate interpreter, effectively bypassing the GIL limitations. - Numba: Numba is a just-in-time (JIT) compiler for Python that specializes in numerical computations. It can compile Python functions to machine code, bypassing the GIL and achieving significant speedups for CPU-bound tasks. Numba integrates well with NumPy arrays and provides a simple way to optimize critical sections of code.
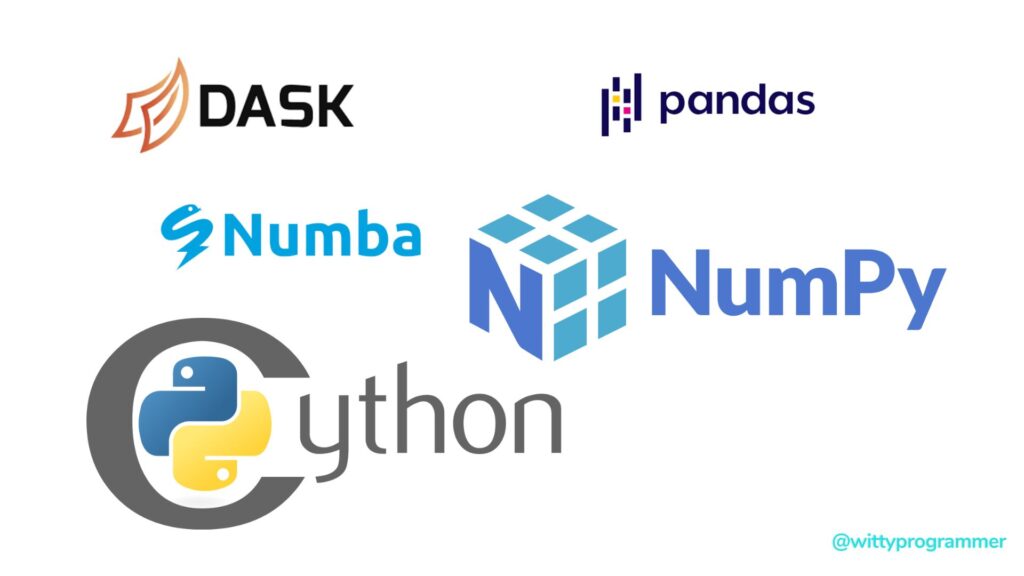
Conclusion
Python multithreading offers convenient concurrency features for managing I/O-bound tasks and improving code organization. While the GIL restricts true parallelism, combining multithreading with asynchronous programming or leveraging it for non CPU-bound tasks can still yield significant performance improvements. By understanding the benefits, limitations, and best practices, you can effectively utilize Python multithreading and develop efficient and responsive applications.
Hope you all have gained proper knowledge about the Python Multi-Threading trends and best practices. Please do share with your friends and like the post if you find it useful. Thank you for reading and keep sharing great work.